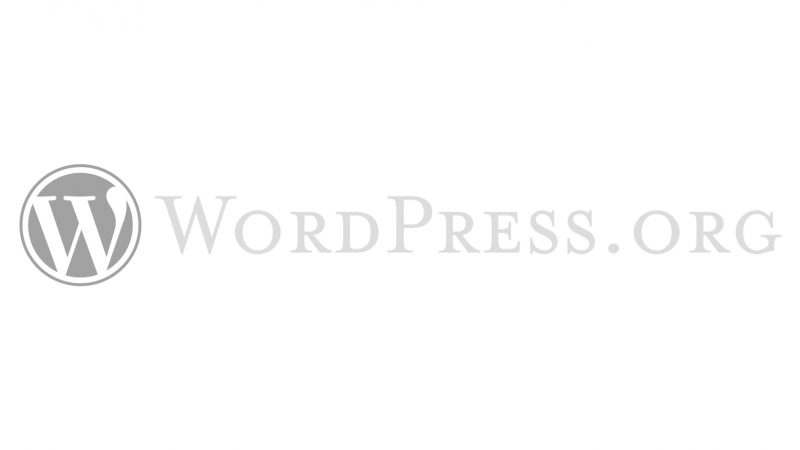
How To Remove Tags & Categories From WordPress Posts
If you’re anything like me, you spend a lot of time building WordPress sites for clients. For the vast majority of these sites, they either don’t require a blog, or the blog is not the main focus of the site. WordPress has a user interface that is generally user friendly, however it’s good to cut back on unnecessary functionality. Post tags are extraneous to the needs of most business blogs and it’s usually best that they be removed. This prevents users being confused by options that aren’t needed. Additionally, tags can be dangerous for SEO if implemented incorrectly.
Removing tags from your WordPress site
Copy the following code into your functions.php
theme file or drop it into your mu-plugins
directory to remove tags.
This code removes tags using the unregister_taxonomy_for_object_type
function (developer reference). This removes all metaboxes and admin pages. However this only covers administrative features, if your posts already have tags applied, they’ll still display on the front end.
Removing tags from the front end
There are a variety of different functions that can be used to retrieve and display the tags for any given post. These functions include:
We can prevent tags from displaying on posts by filtering what these functions return and forcing them to act as though there are no tags.
Preventing tag archives from displaying
Unfortunately all the code provided doesn’t actually remove the tag archives of any existing tags, which still leaves us with those redundant tag archive pages. Removing the links is not enough if you have posts with existing tags, as these tag archives have likely been indexed by search engines already. What we need to do to prevent archive pages from still working is to hijack the main WordPress query and force it to act as though those pages don’t exist.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
add_action( 'pre_get_posts', 'cameronjonesweb_404_tag_archives' ); | |
function cameronjonesweb_404_tag_archives( $query ) { | |
if( $query->is_main_query() && $query->is_tag() ) { | |
$query->set_404(); | |
status_header( 404 ); | |
} | |
} |
Removing categories and other taxonomies
You can replicate this technique for any other taxonomy, such as post categories, or WooCommerce product tags and categories.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
add_action( 'init', 'cameronjonesweb_unregister_categories' ); | |
add_filter( 'get_the_categories', '__return_false' ); | |
add_filter( 'the_category', '__return_false' ); | |
add_filter( 'wp_get_object_terms', 'cameronjonesweb_hide_categories_front_end', 10, 4 ); | |
add_action( 'pre_get_posts', 'cameronjonesweb_404_category_archives' ); | |
/** | |
* Removes categories from blog posts | |
*/ | |
function cameronjonesweb_unregister_categories() { | |
unregister_taxonomy_for_object_type( 'category', 'post' ); | |
} | |
/** | |
* Removes categories from the front end | |
*/ | |
function cameronjonesweb_hide_categories_front_end( $terms, $object_ids, $taxonomies, $args ) { | |
if( in_array( 'category', $taxonomies ) ) { | |
$terms = false; | |
} | |
return $terms; | |
} | |
/** | |
* Remove category pages | |
*/ | |
function cameronjonesweb_404_category_archives( $query ) { | |
if( $query->is_main_query() && $query->is_category() ) { | |
$query->set_404(); | |
status_header( 404 ); | |
} | |
} |
Adding these few lines of code to your WordPress site will make managing your website easier and avoid SEO issues.