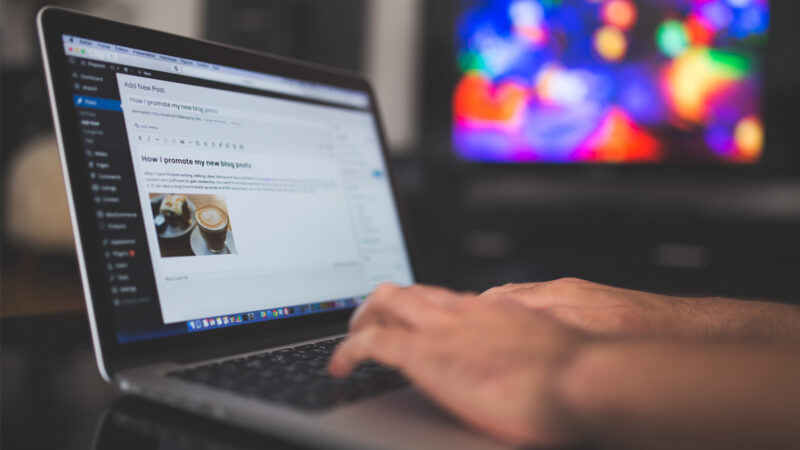
How To Remove The Uncategorised Category From WordPress And WooCommerce
Out of the box, WordPress includes a default category for posts called “Uncategorized”. It not only looks unprofessional, it’s largely unnecessary. While you can rename it to something more suitable, I tend to use “General”, you can’t delete it. At least, not without a bit of code.
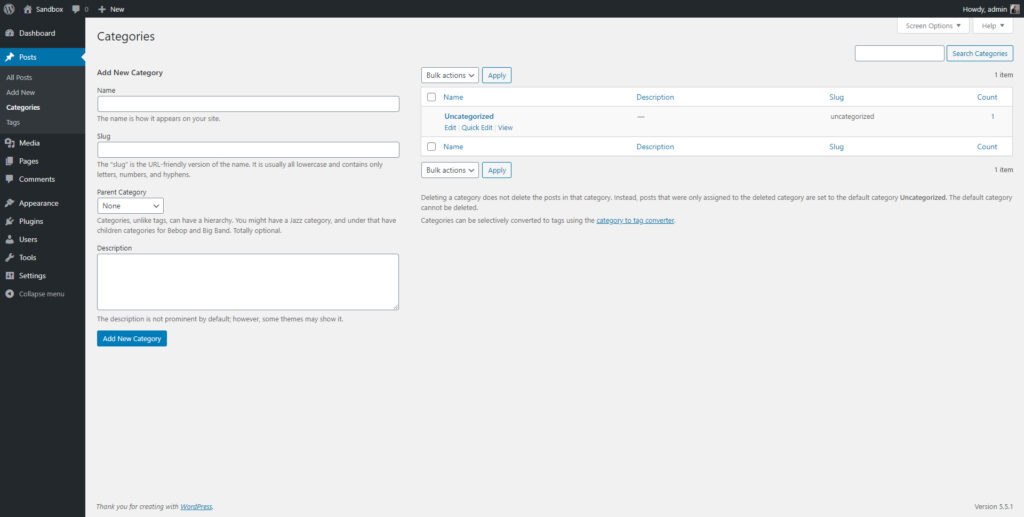
The first thing we’ll want to do is filter the taxonomy arguments, using the register_taxonomy_args
filter. When a taxonomy is registered, using register_taxonomy
, a default term can be provided, so we’ll want to stop WordPress from setting up that default term.
If you were to try to remove the default category at this point, you still won’t be able to. When the default category is initially created, WordPress stores the ID of the category in the options table. So even with us now telling WordPress not to create a default category, because it’s already been created, WordPress still will treat it as the default category. We can use delete_option
to remove that option from the database.
Now that both of those are taken care of, you can go in and delete the default category.
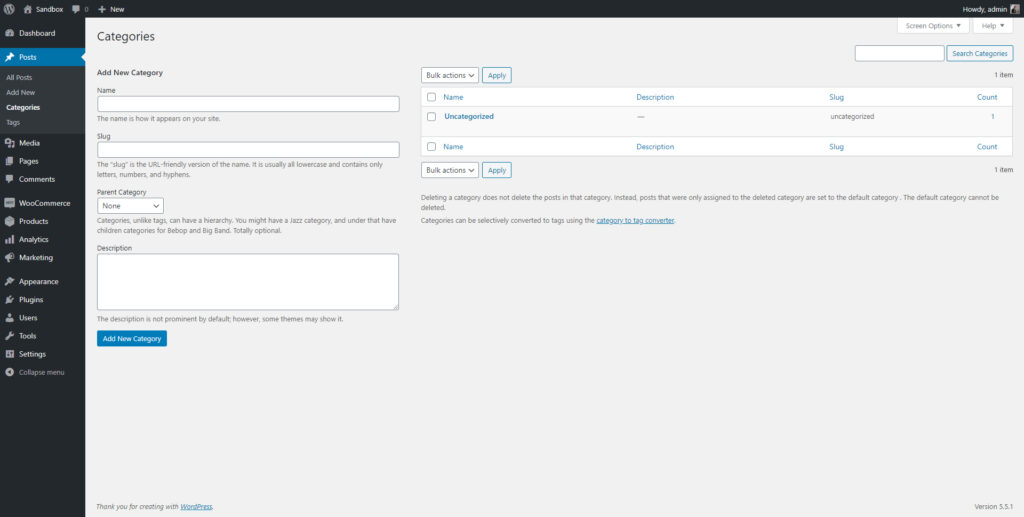
The popular eCommerce plugin WooCommerce also include a default “Uncategorized” category. Again, this is less than ideal. We can extend our code to apply to the WooCommerce product category as well.
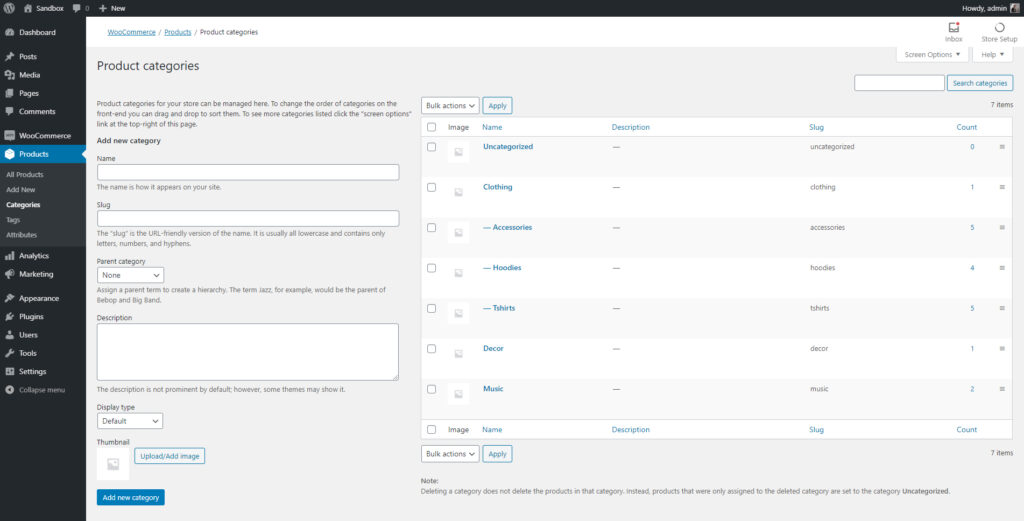
Of course, if there are other plugins that add taxonomies with default terms, you can use the same code to remove their default terms. The one thing to note here is that if you’re modifying a taxonomy registered by a plugin like this, you should add your code as a Must Use plugin. As plugins are loaded before the theme, if the code is in your functions.php
file, every time you update the plugin it will create a new “Uncategorised” term.