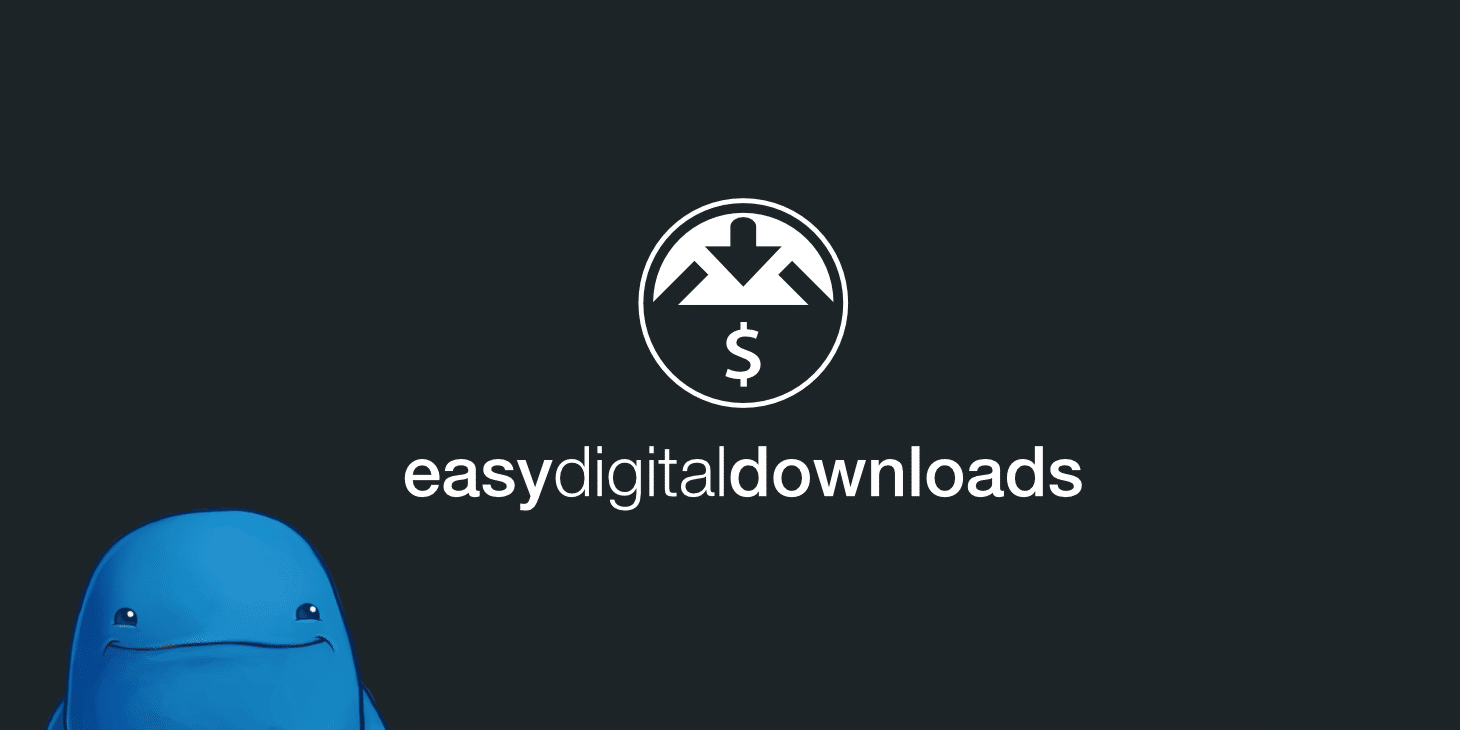
How To Create A Front End Login Page With Easy Digital Downloads
Recently I published a quick tutorial on setting a WooCommerce site to use the WooCommerce login page for all logins instead of wp-login.php
. I wanted to replicate this functionality on a new site I’m building with Easy Digital Downloads.
Easy Digital Downloads requires a bit more work, as by default there’s no option to set a login or account page. But as EDD is a quality plugin it was no surprise that I could easily add a new setting for it using the edd_settings_general
filter. EDD also has a super handy edd_get_option()
function which makes it easy to retrieve the value of our new setting.
On the page that you want to set as your account page make sure you have the [[edd_profile_editor]]
shortcode in the content. The shortcode will display the login form to logged out users, and the profile fields to logged in users.
Once the page and settings are setup, we can pretty much replicate the functionality used for WooCommerce to do the same thing with EDD.
Once you’ve added the code below, your site will automatically redirect any logged out visits to /wp-admin
instead to the new EDD account page, as well as updates any other functions that are displaying a link to the login page.
https://gist.github.com/cameronjonesweb/e609fb74dcb6ab2ad3651c74578043a1#file-edd-account-login-php