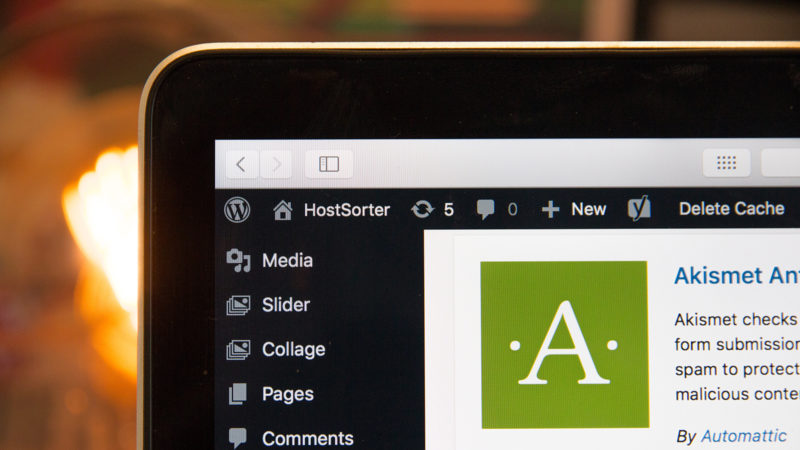
How To Automatically Generate Post Type Labels
WordPress’ custom post types are a fantastic way to organise different types of data. It’s quick and easy to spin up a new post type with just a couple of lines of code. Post types can be used to store anything from products, forms, profiles or even logs, pretty much any type of content stored in a repeatable way.
However one of the more time consuming aspects of creating custom post types is setting all the correct labels. There are dozens of labels used for each post type, all used in different contexts. For the best administrative user experience all the labels should be configured, but often they can be overlooked.
To aid with the creation of post type labels, simply drop this function into your functions.php
file or custom plugin. From there, you can simply call this function, passing through the singular and plural labels, and the rest will be generated for you. And if needed, individual labels can be overwritten.