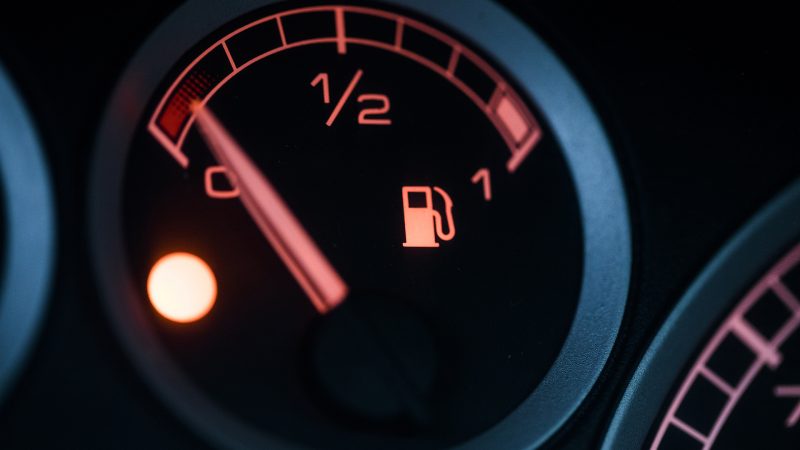
Forcing WP_Query To Return No Results
The WP_Query
object is the primary way of retrieving a post, or a number of posts from a WordPress site. There may be some occasions where you need a WP_Query
object to return an empty result, so that WP_Query::have_posts
returns false
. Some good use cases could be testing for empty results when you’ve already added a lot of test data, or returning empty results because you have some other required user provided parameters that haven’t been specified.
Achieving this is actually pretty easy, all that you need to do is add a post__in
parameter to the WP_Query
with a value of array( 0 )
. What this tries to do is only select posts with an ID of 0
, and as post IDs auto increment, there will never be a post with an ID of 0
, meaning the query will never return any results. This will also work in conjunction with any other parameters passed to the WP_Query
.
https://gist.github.com/cameronjonesweb/722fb8cfa518902fc1f92a868bd0b6ae#file-new-wp-query-php
In the particular instance that I needed to implement this functionality, I needed to return an empty result if someone was on the search results page without putting in a search term (by default, WordPress will return everything if no search term is specified). The implementation here is slightly different but the concept and parameters are the same. When manipulating the main query, you pass in the paramter using an action on the pre_get_posts
hook.
https://gist.github.com/cameronjonesweb/722fb8cfa518902fc1f92a868bd0b6ae#file-pre-get-posts-filter-php